Functions are the building blocks of JavaScript basics, allowing developers to organize and structure their code efficiently.They are one of the fundamental concepts in programming. In this article, you will learn what functions are in JavaScript, how to write your own custom functions, and how to implement them effectively in your code.
Javascript basics :
JavaScript, the programming language that powers the dynamic content on the web, relies heavily on functions of javascript basics.
What are Functions in JavaScript?
A function in JavaScript basics is a set of statements that take inputs, do some specific computation, and produce output. A function in JavaScript is similar to a procedure— statements that perform a task, but for a procedure to qualify as a function. The function consists of one statement that says to return the parameter of the function (that is, number) multiplied by itself.
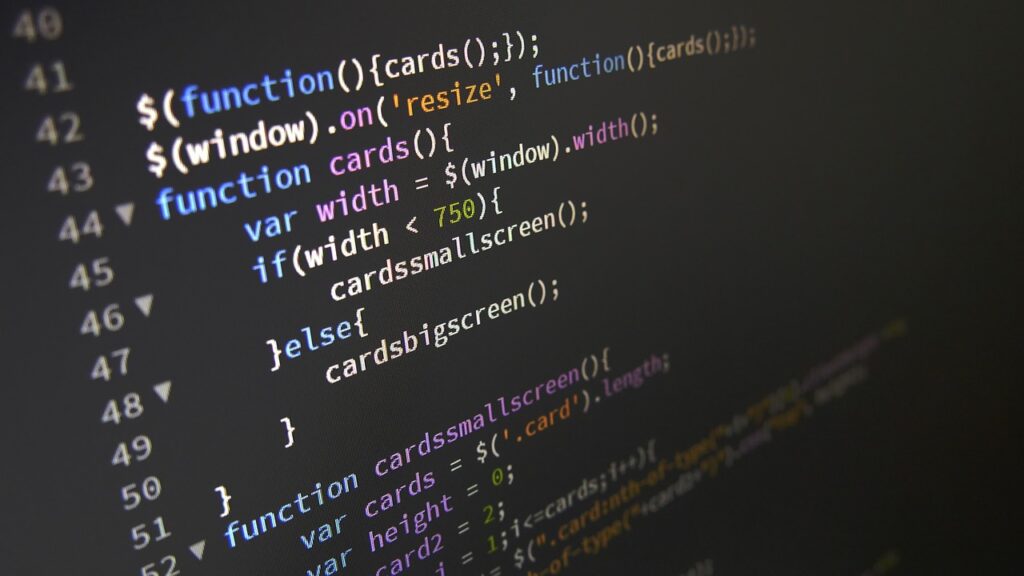
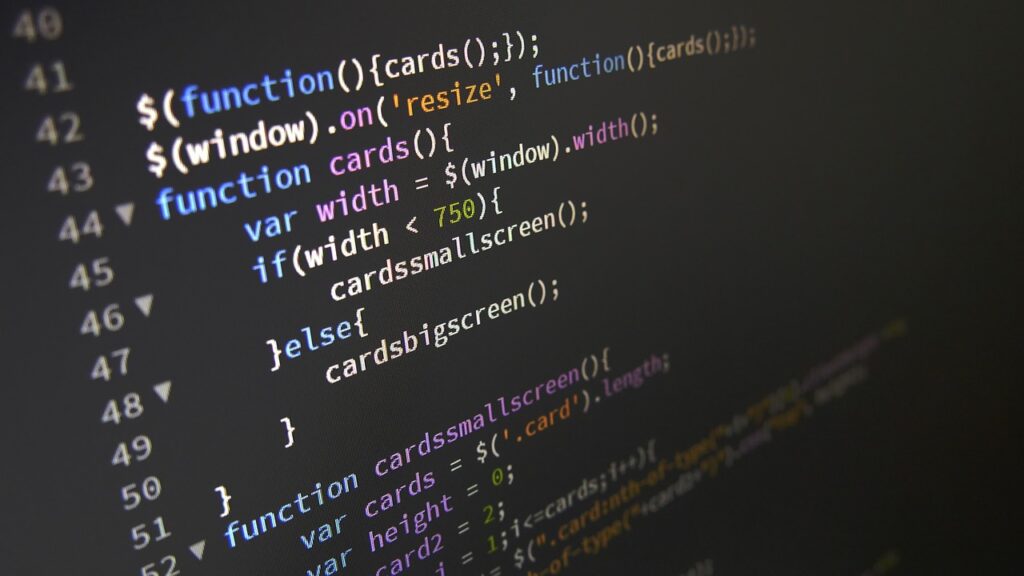
1. The fundamental of Functions in Javascript basics
1.1 Function Declaration and Invocation
A function is a reusable block of code which performs a specific task. You can declare a function using the function keyword, followed by the function name and a pair of parentheses.
function greet(name) {
console.log(`Hello, ${name}!`);
}
To invoke or call the function, use its name followed by parentheses and any necessary arguments:
greet("John"); // Outputs: Hello, John!
1.2 Function Parameters and Return Values
Functions can accept parameters, which act as variables within the function body. These parameters allow you to pass values to the function when calling it. Additionally, functions can return values .
function add(a, b) {
return a + b;
}
const result = add(3, 5);
console.log(result); // Outputs: 8
1.3 Function Expressions
Another way to define functions is through function expressions. In this approach, you assign an anonymous function to a variable:
const multiply = function(x, y) {
return x * y;
};
console.log(multiply(4, 6)); // Outputs: 24
1.4 Function Declaration
function greet(name) {
return `Hello, ${name}!`;
}
1.5 Arrow Functions
ES6 introduced arrow functions, a concise syntax for writing functions, especially useful for short, one-line functions:
const square = (num) => num * num;
console.log(square(9)); // Outputs: 81
Click to read the latest blog on Javascript basics- Javascript and its best traits.
2. Scope and Closure of Javascript basics
Understanding scope is crucial when working with functions in JavaScript basics. It has function-level scope, meaning variables defined inside a function are not accessible outside, and vice versa. However, ES6 introduced the let and const keywords, enabling block-level scope.
2.1 Global Scope
Variables declared outside any function or block have a global scope, making them accessible throughout the entire program. Be cautious with global variables, as they can lead to naming conflicts and unintended side effects.
const globalVar = "I am global";
function printGlobalVar() {
console.log(globalVar);
}
printGlobalVar(); // Outputs: I am global
2.2 Closure
Closure occurs when a function retains access to variables from its outer (enclosing) scope, even after the outer function has finished executing. This can be a powerful concept in JavaScript for creating modular and encapsulated code:
function outer() {
const outerVar = "I am outer";
function inner() {
console.log(outerVar);
}
return inner;
}
const closureFunction = outer();
closureFunction(); // Outputs: I am outer
3. First-Class Functions and Higher-Order Functions
JavaScript treats functions as first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, and returned as values from other functions.
3.1 Function as Variables
You can assign functions to variables and use them as any other variable type:
const sayHello = function(name) {
console.log(`Hello, ${name}!`);
};
sayHello("Alice"); // Outputs: Hello, Alice!
3.2 Function as Parameters
Functions can take other functions as arguments, allowing for dynamic and flexible behavior. This is a key concept in higher-order functions:
function operateOnNumbers(a, b, operation) {
return operation(a, b);
}
const addNumbers = (x, y) => x + y;
const multiplyNumbers = (x, y) => x * y;
console.log(operateOnNumbers(3, 4, addNumbers)); // Outputs: 7
console.log(operateOnNumbers(3, 4, multiplyNumbers)); // Outputs:12
3.3 Function as Return Values
Functions can also return other functions, leading to the concept of higher-order functions:
function greetFactory(greeting) {
return function(name) {
console.log(`${greeting}, ${name}!`);
};
}
const sayHi = greetFactory("Hi");
sayHi("Bob"); // Outputs: Hi, Bob!
4. Recursion in Functions
It is a technique in which a function calls itself. It’s a powerful concept for solving problems that can be broken down into smaller, similar sub-problems.
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
console.log(factorial(5)); // Outputs: 120
However, it’s essential to handle recursion carefully to avoid infinite loops and excessive memory usage.
5. Callbacks, Promises, and Async/Await- Javascript basics
JavaScript’s asynchronous nature is often managed using callbacks, promises, and async/await. Understanding these concepts is crucial for dealing with asynchronous code effectively.
5.1 Callbacks
A callback is a function passed as an argument to another function, to be executed later:
function fetchData(callback) {
// Simulating asynchronous operation
setTimeout(() => {
const data = "Fetched data";
callback(data);
}, 1000);
}
function processFetchedData(data) {
console.log(`Processing: ${data}`);
}
fetchData(processFetchedData); // Outputs: Processing: Fetched data
5.2 Async/Await
Async/await is a syntactic sugar built on top of promises, providing a more readable and concise way to write asynchronous code:
async function fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
const data = "Fetched data";
resolve(data);
}, 1000);
});
}
async function fetchDataAndProcess() {
try {
const data = await fetchData();
console.log(`Processing: ${data}`);
} catch (error) {
console.error(`Error: ${error}`);
}
}
fetchDataAndProcess(); // Outputs: Processing: Fetched data
5.4 Higher-Order Functions
JavaScript treats functions as first-class citizens, allowing them to be passed as arguments to other functions and returned as values from other functions.
const multiplyBy = (factor) => (num) => num * factor;
const double = multiplyBy(2);
console.log(double(5)); // Output: 10
In this example, multiplyBy is a higher-order function that returns another function. This enables the creation of more flexible and reusable code.
Best Practices and Tips
Understanding functions in JavaScript and javascript basics are essential for writing efficient and maintainable code. To solidify your knowledge and apply best practices, consider the following tips and practices:
Click to read the trendy blog about workflow automation-Positive effects of workflow automation.
1. Master the Javascript Basics:
Before diving into advanced concepts, ensure a strong grasp of the basics. Understand how to declare functions, pass parameters, and use the return statement. Practice writing simple functions to reinforce your understanding.
2. Embrace Function Expressions:
Get comfortable with both function declarations and expressions. Function expressions, especially arrow functions, offer a concise syntax and are commonly used in modern JavaScript code.
3. Name Your Functions Descriptively:
Choose meaningful and descriptive names for your functions. This makes your code self-explanatory, enhancing readability and making it easier for collaborators to understand your intentions.
4. Use Parameters Wisely:
Be mindful of the number and type of parameters your functions accept. Aim for simplicity and avoid excessive parameters. If a function requires many inputs, consider grouping related parameters into an object.
5. Return Explicitly:
Always use the return statement to explicitly return values from your functions. This promotes clarity and ensures that the function’s output is well-defined. Avoid relying on implicit returns.
6. Understand Higher-Order Functions:
Explore higher-order functions, as they can greatly enhance the flexibility and expressiveness of your code. Learn to use functions as arguments and return functions when necessary.
7. Become Proficient with Callbacks:
Callback functions are prevalent in asynchronous JavaScript. Understand how to use them effectively, especially when working with events, timers, or making asynchronous requests.
8. Explore Arrow Functions:
Familiarize yourself with arrow functions and their benefits, such as lexical scoping of this. Use arrow functions when concise syntax is suitable, especially in cases like mapping and filtering arrays.
9. Grasp Closures:
Understand the concept of closures, as they can be powerful and sometimes unexpected. Recognize scenarios where closures are beneficial, such as creating private variables or maintaining state between function calls.
10. Test Your Functions:
Practice writing tests for your functions using testing frameworks like Jest or Mocha. Testing ensures that your functions behave as expected and facilitates code maintenance and refactoring.
11. Avoid Global Variables:
Minimize the use of global variables within your functions to prevent unintended side effects. Opt for local variables and pass data through parameters or return values.
12. Documentation is Key:
Document your functions using comments or JSDoc annotations. Clearly describe the purpose of the function, expected inputs, and the format of the return value. Good documentation helps others (and yourself) understand your code.
13. Stay Updated on ECMAScript Features:
JavaScript evolves, and new features are regularly introduced. Stay informed about the latest ECMAScript specifications to leverage new language features and best practices.
14. Practice, Practice, Practice:
The more you practice writing functions, the more confident and proficient you’ll become. Work on small projects, solve coding challenges, and review existing codebases to reinforce your skills.
15. Use Descriptive Names
Choose meaningful names for your functions. A descriptive name makes your code more readable and self-explanatory.
// Good
function calculateArea(radius) {
return Math.PI * radius * radius;
}
// Bad
function calcAra(r) {
return Math.PI * r * r;
}
16. Keep Functions Small and Single-Purpose
Functions should ideally perform a single, well-defined task. This promotes code readability, reusability, and easier maintenance.
17. Avoid Side Effects
A function should not modify external state or produce side effects. Side-effect-free functions are easier to reason about and test.
18. Default Parameters and Destructuring
Utilize ES6 features like default parameters and destructuring to enhance function flexibility.
function greet({ name = 'User', greeting = 'Hello' }) {
return `${greeting}, ${name}!`;
}
console.log(greet({ name: 'John', greeting: 'Hi' })); // Output: Hi, John!
Conclusion
Functions are fundamental to JavaScript and javascript basics, serving as the building blocks for creating modular, reusable, and maintainable code. From basic function declarations to advanced concepts like closure, higher-order functions, recursion, and asynchronous programming, a solid understanding of functions is essential for any JavaScript developer.
As you continue to explore JavaScript and javascript basics, practice implementing functions in various scenarios. Experiment with different function types, scopes, and asynchronous patterns to deepen your understanding. The mastery of functions will empower you to create efficient, scalable, and well-organized JavaScript applications.