Design patterns play a critical role in software development, guiding developers on how to structure their code to achieve optimal efficiency, scalability, and maintainability. In this guide, we will explore the definition and importance of it, as well as debunk common misconceptions surrounding their usage.
What are design Patterns?
Design patterns are the solution to the problems that arise for the coders in making the software. It help developers create flexible, modular, and scalable software by providing a set of best practices for solving common design problems.
They can be categorized as creational, structural, and behavioral. Creational patterns focus on mechanisms of object creation, structural patterns deal with object composition, and behavioral patterns address how objects interact with each other. Each category contains several individual patterns that can be applied to different aspects of software development.
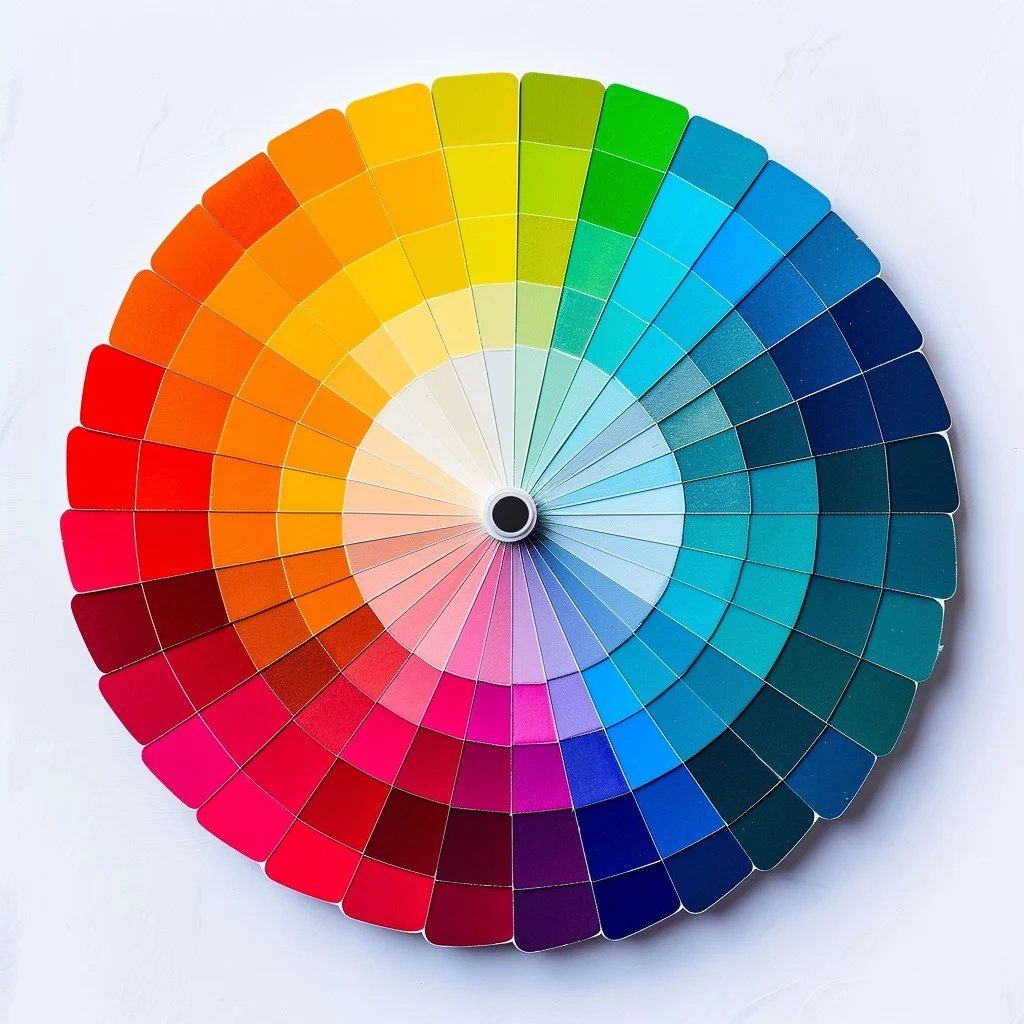
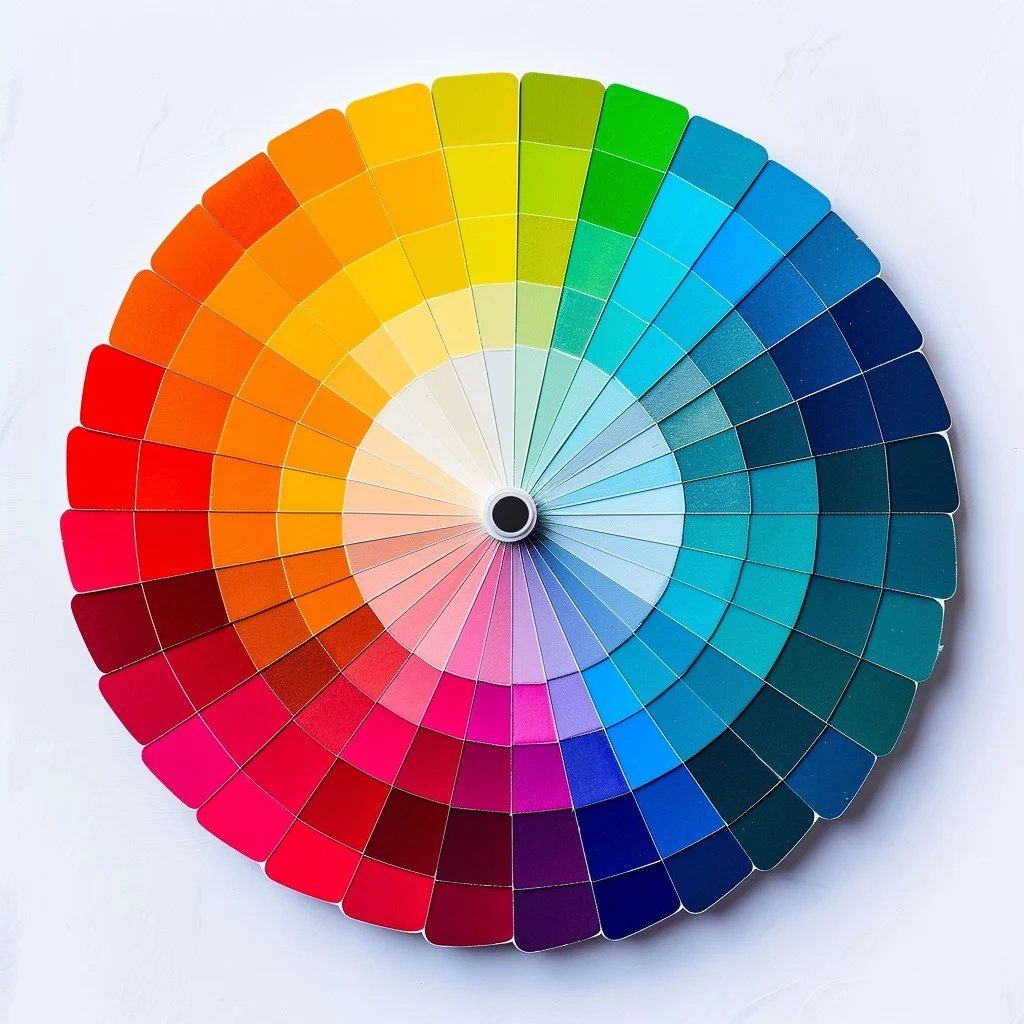
Importance of Design Patterns
The significance of design patterns is that they promote code reusability. By adopting these established patterns, developers can avoid reinventing the wheel and leverage proven solutions to common problems. This also saves time for the coders and makes work easy.
Why Software Design is a Best Practice?
Because Software design patterns have various advantages when it comes to designing and implementing code. Firstly, they improve the maintenance ability of the codebase. By following well-defined patterns, the code becomes easier to read, understand, and modify.
1. Reusability: Design patterns provide a set of proven solutions to common design problems, allowing developers to reuse these solutions in different contexts. This saves time and effort.
2. Maintainability: By following established design, developers can create code that is easier to maintain and update, as the structure and organization of the code are well-defined and consistent.
3. Scalability: As software systems grow in complexity, it becomes crucial to design code in a way that allows it to adapt to changing requirements. It also help to create flexible and scalable software architecture, making it easier to add new features or modify existing ones without impacting the entire system.
4. Extensibility: By separating different aspects of the codebase into distinct components, patterns allow for easy integration of new functionalities.
5. Collaboration: The style provides a common language and framework for communication among developers, making it easier for team members to understand and work on each other’s code.
Click to read our fresh article on Application security best practices.
Common design in programming
Creational plan:
Creational ones are a category of a pattern in software development that deal with the creation of objects. These patterns provide ways to create objects in a manner that is flexible, efficient, and reusable.
Factory Method:
The Factory Method pattern allows for the creation of objects without specifying their concrete classes. It provides an interface for creating objects but leaves the actual instantiation to subclasses.
Abstract Factory Pattern:
By using this, you can easily switch between different families of objects without modifying the client code. It promotes loose coupling and helps in creating a flexible and extensible system.
Singleton Pattern:
This is quite useful when you have resources that need to be shared across different parts of your application and you want to ensure that there is only one instance of the class managing those resources.
Prototype Pattern:
Creates new objects by copying an existing object, known as the prototype, rather than creating new instances from scratch.
Builder Pattern:
This is great when you have objects with many configurable options or when you want to separate the construction logic from the actual object creation.
Structural Pattern:
Structural design are a set of well-defined techniques that help developers organize and structure their code in a scalable and maintainable way. These patterns deal with the relationships between objects, helping to define how they can interact and work together to achieve a common goal.
- Adapter Pattern: This pattern allows objects with incompatible interfaces to work together by creating a middle Adapter class that translates requests from one object to another.
- Proxy Pattern: The Proxy pattern provides a surrogate or placeholder for another object to control access to it. It can be used to add additional functionality to an object or control its usage, without the client knowing the difference.
- Decorator Pattern: This pattern allows behavior to be added to an object dynamically, by wrapping the object in another class that provides additional functionality.
- Bridge Pattern: The Bridge pattern decouples an abstraction from its implementation, allowing it to vary independently. It uses an interface or abstract class as a bridge between the abstraction and its implementations.
- Composite Pattern: This pattern provides a way to treat a group of objects (a composite) in the same way as individual objects. It creates a tree-like structure to represent part-whole hierarchies.
- Facade Pattern: This pattern provides a simplified interface to a complex subsystem, making it easier to use. It encapsulates the interactions and provides a higher-level interface that is easier to use and understand.
- Flyweight Pattern: This pattern reduces memory usage by sharing common data across multiple objects. This reduces memory consumption and improves performance.
3. Behavioral Design Patterns:
They focus on the interaction and communication between objects and classes in a system. They help to define the behavior and responsibilities of the objects and how they collaborate to achieve a certain goal. Some common design patterns includes:
- Observer Pattern: This pattern establishes a one-to-many relationship between objects, where changes in one object trigger updates in its dependent objects.
- Strategy Pattern: The strategy pattern allows you to define a family of algorithms, encapsulate them separately, and make them interchangeable at runtime.
- State Pattern: The state pattern allows an object to alter its behavior when its internal state changes. It helps manage complex state transitions in a clean and easy-to-understand way.
- Interpreter Pattern: The Interpreter design pattern is a behavioral design pattern that enables defining a language, evaluating the sentences in the language, and executing code based on the input.
- Command Pattern: The command pattern encapsulates a request as an object, allowing you to parameterize clients with queues, requests, or operations. It decouples the sender and receiver of a command.
- Chain of Responsibility Pattern: The Chain of Responsibility design pattern is a behavioral pattern that allows an object to pass a request along a chain of potential handlers until one of them handles the request.
- Mediator Pattern: The mediator design pattern is a behavioral design pattern that promotes loose coupling between objects by encapsulating the way they communicate with each other. In this pattern, a mediator object acts as a middleman between different elements (colleagues) and handles their interactions.
- Memento Pattern: The Memento pattern is a behavioral design pattern that captures and stores the internal state of an object in a way that allows it to be restored later, without violating encapsulation.
- Template Pattern: The template method design pattern is a behavioral pattern that defines the outline of an algorithm but allows subclasses to customize certain steps of the algorithm without changing its structure.
- Visitor Pattern: The visitor method design pattern is a behavioral design pattern that allows you to separate the algorithms from the objects on which they operate.
Implementing Design Pattern Tutorial:
Above image is the code in python for applying the “singleton pattern”.
Object – Oriented Design Strategies:
Object-oriented design strategies are techniques used to plan and develop software systems using object-oriented programming principles. Some of the strategies are:
1. Encapsulation: This strategy involves packaging related data and behavior into objects, allowing objects to interact with each other.
2. Inheritance: Inheritance lets you define new classes by extending existing ones.
3. Polymorphism: This strategy helps achieve code flexibility by allowing objects to assume different forms based on the context in which they’re used.
4. Abstraction: Abstraction involves defining simple yet representative structures to model complex systems.
5. Composition: Composition promotes building complex objects by combining simpler ones.
6. Interface-based design: Interfaces define a common set of methods that implementing classes must adhere to.
Conclusion
Design patterns play a crucial role in software development as they enable the creation of robust, reusable, and maintainable code. They provide a common language and framework that developers can rely on to solve recurring problems efficiently. “We can use these software design patterns in different languages like in C++, python, javascript, java etc.”