JavaScript is a popular and versatile programming language that powers the dynamic and interactive features of many websites and applications. JavaScript, often hailed as the “language of the web,” One of the fundamental aspects of JavaScript programming is understanding variables and data types. These concepts serve as the building blocks for any JavaScript application.
In this article, we will learn about some of the basic concepts of JavaScript, what variables are, the various data types in JavaScript, and how to use them effectively in your code.
What are variables?
Variables is a container for storing data values that can be used and manipulated in a program. Variables have names that identify them and can be assigned different values using the = operator. For example, we can create a variable named greeting and assign it the value “Hello”:
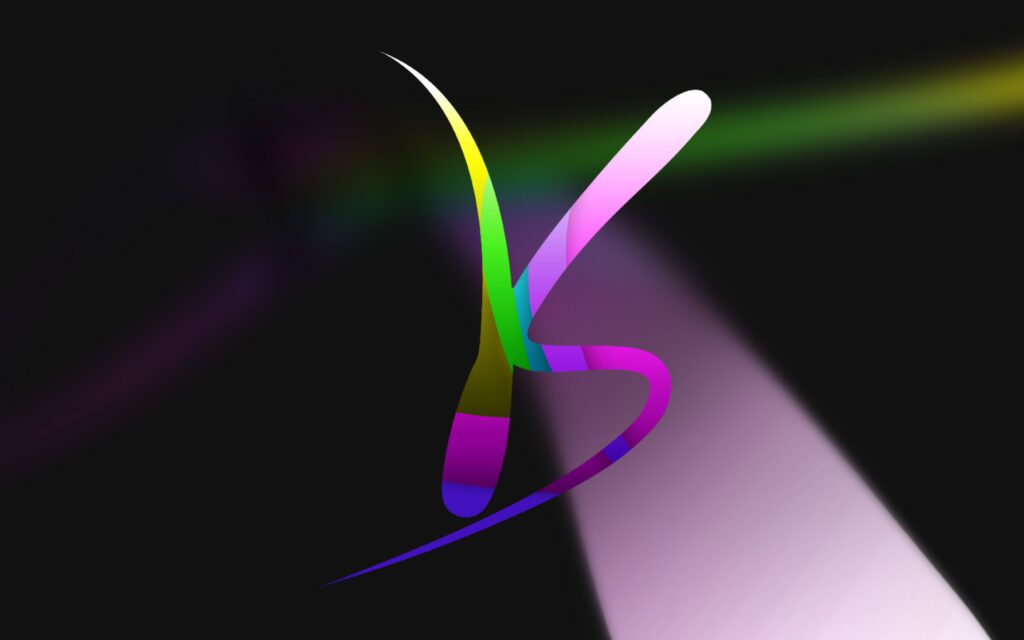
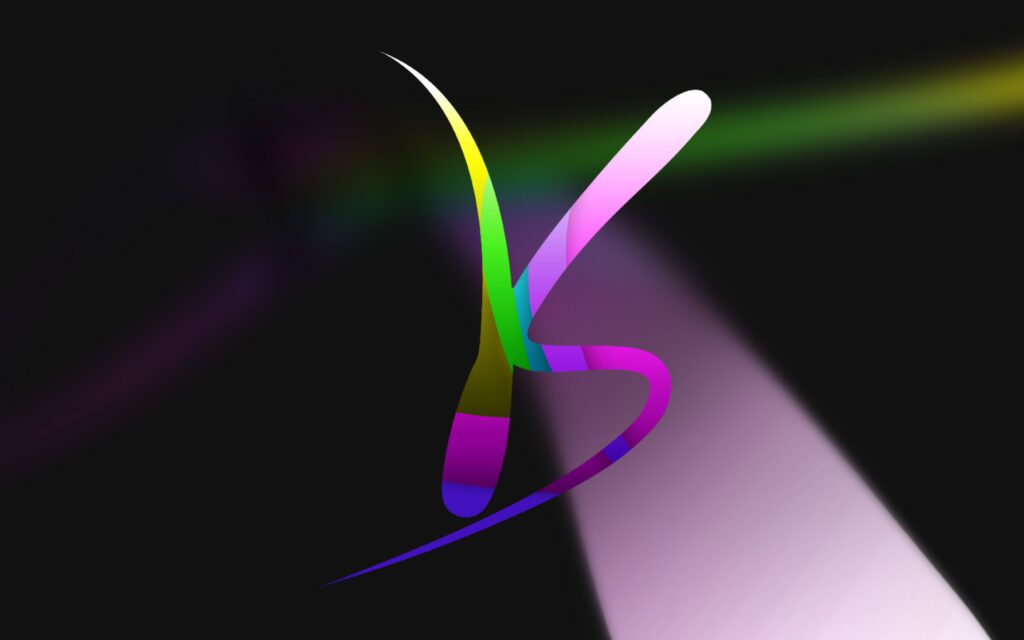
Understanding Variables
Variables are containers that store data values. In JavaScript, you can think of a variable as a labeled box in which you can store information. These boxes can be used to hold different types of data, such as numbers, strings, or even more complex structures like arrays and objects.
Check out the latest article on DOM basic values- JS in DOM manipulation.
What are data types?
Data types are categories that classify the values that variables can hold. Different data types have contrasting properties and behaviors. JavaScript has several built-in data types, such as:
String: A sequence of characters enclosed in quotes, such as “Hello” or ‘Hi’. Strings are used to represent text or symbols.
Number: A numeric value, such as 42 or 3.14. Numbers can be used for arithmetic operations, such as addition, subtraction, multiplication, and division.
Boolean: Booleans represent either true or false values. Booleans are used for conditional statements, such as if or while.
Undefined: A special value that indicates the absence of a value.
Null: A special value that represents an intentional absence of a value. Null is different from undefined, as it implies that the variable has been assigned a value, but that value is null.
Object: A collection of properties and methods that define the state and behavior of a complex entity. Objects can have other data types as their properties or values. For example, an object can represent a person, with properties such as name, age, and gender, and methods such as greet() or sayHello().
Array: A special type of object that stores multiple values in a single variable. Arrays are ordered and indexed, meaning that each value has a position and a number associated with it. For example, an array can store a list of fruits, such as [“apple”, “banana”, “orange”].
Declaring Variables
To use a variable in JavaScript, you need to declare it using the ‘var’, ‘let’, or ‘const’ keyword, followed by the variable name.
Here’s a brief overview of each:
- var: Historically used for variable declaration, but it has some scoping issues and is now mostly replaced by ‘let’ and ‘const’.
var age = 25;
- let: Introduced in ECMAScript 6 (ES6), ‘let’ allows you to declare variables with block scope, making it more predictable and manageable.
let name = "John";
- const: Also introduced in ES6, ‘const’ is used to declare constants, variables whose values should not be changed once assigned.
const pi = 3.14;
Variable Naming Conventions
When naming variables, it’s essential to follow certain conventions for readability and maintainability. Variable names should be descriptive, start with a letter, and use camelCase for multi-word names.
For example:
let myVariable = "Hello, World!";
let userAge = 30;
JavaScript Data Types
JavaScript is a dynamically typed language, meaning you don’t need to explicitly specify a data type when declaring a variable. The interpreter determines the type dynamically based on the value assigned.
Types of some basic data types in JavaScript:
1. Primitive Data Types
a. String
The string data type represents sequences of characters surrounded in single or double quotes.
let greeting = "Hello, World!";
let company = 'ABC Corp'’
b. Number
The number data type represents integers and floating-point numbers.
let age = 25;
let price = 19.99;
c. Boolean
Booleans represent either ‘true’ or ‘false’ values.
let isStudent = true;
let hasJob = false;
d. Undefined
When a variable is declared but not allot a value, it is undefined.
let undefinedVariable;
console.log(undefinedVariable); // Outputs: undefined
e. Null
Null represents data type and represents the absence of a value.
let nullValue = null;
f. Symbols (ES6+)
Symbols are unique and immutable data types introduced in ES6. They are often used as property keys in objects to prevent name collisions.
const mySymbol = Symbol('description');
Click to read our insightful blog on programming updates- Effective strategies of staying updated.
2. Complex Data Types
a. Array
Arrays are ordered lists that can hold elements of different data types.
let fruits = ["apple", "banana", "orange"];
let mixedArray = [1, "two", true];
b. Object
Objects are collections of key-value pairs and are used to represent entities.
let person = {
name: "John",
age: 30,
isStudent: false
};
c. Function
Functions are blocks of reusable code that can be defined .
function greet(name) {
console. log.("Hello, " + name + "!");
}
greet("Alice"); // Outputs: Hello, Alice!
Type Conversion
JavaScript also allows you to convert between data types. For example, you can change a string to a number or vice versa.
let numString = "42";
let num = Number(numString);
let age = 25;
let ageString = age.toString();
Best Practices and Common issues
1. Variable Scope
Understanding variable scope is crucial in JavaScript. Variables declared with ‘var’ have function scope, while those declared with ‘let’ and ‘const’ have block scope. This affects where the variable is accessible within your code.
function example() {
if (true) {
var x = 10; // Function-scoped
let y = 20; // Block-scoped
}
console.log(x); // Outputs: 10
console.log(y); // Error: y is not defined
}
2. NaN and Type Coercion
Be cautious when performing operations that involve different data types. JavaScript may perform type coercion, converting one type to another.
let result = "5" * 2; // Result is 10 (string converted to number)
let invalidResult = "Hello" - 3; // Result is NaN (not a number)
Conclusion
Variables and data types are fundamental concepts in JavaScript programming. By understanding how to declare variables and work with different data types, you can effectively store and manipulate data within your JavaScript programs. Consider to choose the suitable data type for your variables based on the type of data you need to work with, and be mindful of type conversion to avoid unexpected behavior in your code. With practice and experimentation, you’ll become proficient in using variables and data types to build powerful and dynamic JavaScript applications.