JSON in Javascript
JavaScript Object Nation or JSON for short is a simple tool used to format data. This tool uses key-value pairs and each key is a string attached to a value. These values are encompassed by numbers, arrays, booleans, strings, and other JSON objects. It is lightweight and easy to read and write for us humans, meanwhile, machines can efficiently analyse and generate it. In simple terms, it is a subset of JavaScript which is supported by many programming languages and it is commonly used in data exchange between servers and web applications.
JSON in JavaScript applications is often to encode and transmit data between servers and their respective clients. While sending and receiving information from APIs, it is often done through HTTP responses and responses between the server and the client. This article will serve as a tutorial and explain the processes of parsing and stringing JSON in JavaScript, both of which are essential operations for working with data transmission across networks. We will dive into the basics of JSON along with parsing and stringing JavaScript data into JSON data. Moreover, for a deeper understanding of the processes, we will look at real-world examples of JSON strings used in web development.
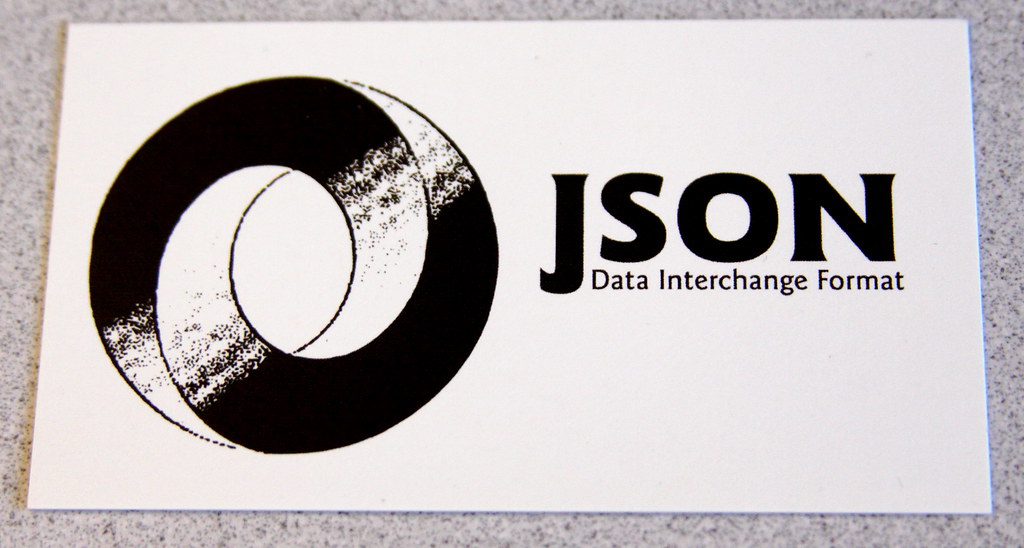
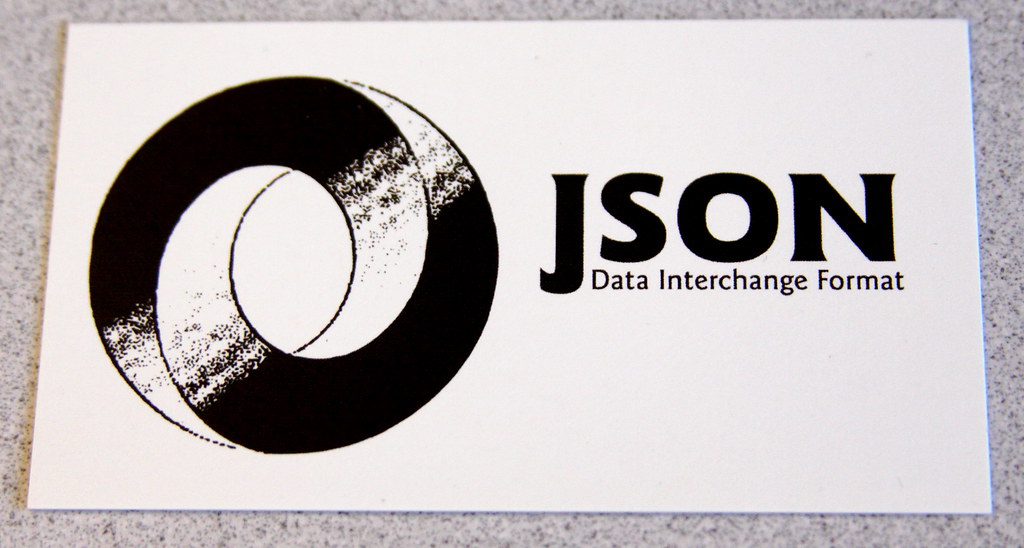
Parsing and stringifying data
Since JSON uses a text-based format, parsing and stringifying are essential to convert raw JSON data to JavaScript objects and vice versa. To do this, we can use the in-built JSON.parse() tool in JavaScript. This method takes JSON strings as inputs and renders them as Javascript objects. Stringifying JSON in JavaScript involves converting a JavaScript object into JSON strings which can be used or stored as a text. This process is facilitated by using the built-in JSON.stringify() tool. This tool takes JavaScript objects as inputs and reproduces them as JSON strings. To sum it up, Parsing involves converting JSON data into JavaScript objects while Stringifying involves converting JSON objects into JavaScript data.
Click to read our latest article on CSS fundamentals- CSS fundamental text and font styling.
Parsing JSON in Javascript
To further understand the concept of Parsing, we will be looking at the concept through a relevant example.
const jsonString = '{"name": "John Doe", "age": 30, "isStudent": false}';
const person = JSON.parse(jsonString);
console.log(person.name); // Output: John Doe
console.log(person.age); // Output: 30
console.log(person.isStudent); // Output: false
In this case, we will be defining a JSON String named ‘jsonString’. This string consists of key-value pairs that contain information about a person’s name, age, and occupation. We then call upon the JSON.parse() method and pass the JSON String as an argument. This object will then be taken in as input and returned as a String.
It is important to note that the JSON String must be well-formed with proper syntax to conform to the JSON format. If the String is not in proper format, the system will show an error. It is important to deal with errors that may occur during the parsing process. Just like normal JSON structures, Nested JSON structures can also be handled in the same way. The JSON.parse() can handle complex JSON structures including arrays and nested objects. However, to fully comprehend the complex data structures, one needs to have an in-depth understanding of the same.
Learn our Calanjiyam artcile on JS strings and more- Salient actions of JS strings.
Stringifying JSON in Javascript
The Sringifying of JavaScript objects into JSON strings can be easily done by the built-in JSON.stringify() command. This method takes JavaScript objects as inputs and produces JSON Strings as outputs. We will demonstrate the Stringify command through an example
const person = {
name: 'John Doe',
age: 30,
isStudent: false,
};
const jsonString = JSON.stringify(person);
console.log(jsonString);
In this example, we first define the JavaScript object called person, which in this case has the same key-value pairs as used earlier during the parsing method. JSON.stringify()` offers the flexibility to tailor the output, enabling the inclusion/exclusion of properties and specifying indentation levels. For instance:
const circularReference = { value: 42 };
circularReference.myself = circularReference;
console.log(JSON.stringify(circularReference));
const jsonString = JSON.stringify(circularReference, (key, value) => {
if (key !== 'myself') {
return value;
}
});
console.log(jsonString);
Practical Applications of JSON in Javascript:
The significance of parsing and stringifying JSON transcends theoretical understanding and finds its essence in practical applications within web development:
1. Seamless API Communication of JSON in Javascript-
Web APIs predominantly communicate using JSON. The ability to parse JSON empowers web applications to comprehend API responses, while stringifying facilitates the transmission of data to the server.
2. Persistent Local Storage-
JSON’s versatility shines through in the realm of local storage within web browsers. By stringifying data, it can be stored persistently and subsequently parsed to retrieve it as needed.
3. Dynamic Configuration Files-
JSON serves as the cornerstone for client-side configuration files, owing to its simplicity and readability. By parsing these files, applications can dynamically access and utilize configuration settings.
Conclusion
In summation, parsing and stringifying JSON in Javascript stand as indispensable operations within the realm of web development. JSON’s standardized format facilitates seamless data exchange and interoperability across diverse systems. Whether navigating through API interactions, orchestrating local data storage, or dynamically configuring applications, a profound understanding of JSON parsing and stringifying empowers developers to navigate the intricacies of data management effectively. Through mastering these operations, developers unlock the full potential of JSON, fostering a seamless and robust data interchange ecosystem on the web.
.