In programming languages, there are various variables and data types in programming by which computers store the provided data in their memory. We will learn about the programming variables and data types in this article. During the communication between humans and computers, humans provide the inputs so that computers can store them.
What are variables?
In programming, variables are the symbolic representation of numbers, where any number we give in the input is represented as an alphabet. They are like holder that holds various information. While creating a variable you provide a name and have to assign a value or can change the value and use it for calculations or comparisons. Let’s see some common types of variables.
For example a=5. This way the computer stores the value of alphabet a as 5 which may vary.
What are data types?
In programming data types define the type of a value. Different programming languages may have their own specific data types. They perform various operations on data and determine how data is stored in the computer’s memory. Let us see some common types of data types.
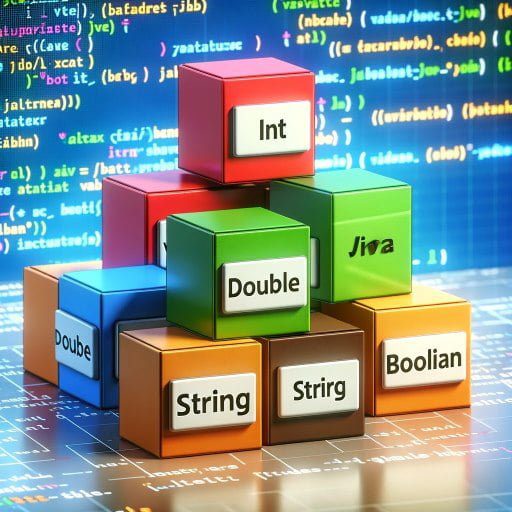
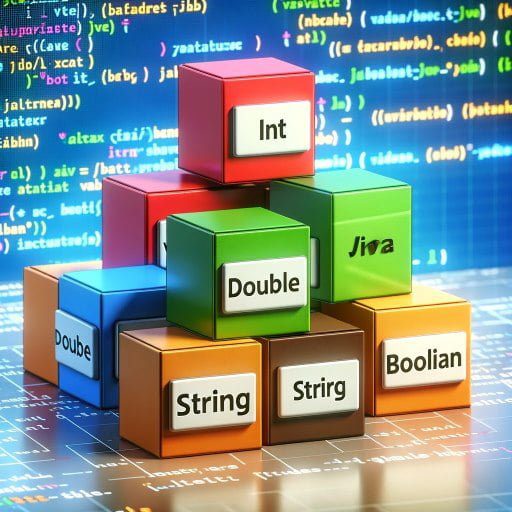
Programming data types
In programming variables and data types, data types are classified into several types based on the data type they can hold. Here we can learn some common types of variables in coding that we use,
Integer
This programming data type can store values like numbers that can be both negative and positive and also zero.
Example: -3,-6,0,1.8 etc.
Floating-points
Here the programming data type, numbers are in decimal values.
Example: 2.54,1.8,0.0007 etc.
Character
Here the data type that stores single characters either numbers or alphabets. Each character is represented within a single quotation
Example: ‘k’, ‘a’,’7’,’3’ etc
String
This programming data type stores a sequence of characters like words or sequences of alphabets. Each sequence of characters is represented in double quotations.
Example: “hello” etc
Boolean
A boolean programming data type is a data type that can only have one of two possible values: true or false. In many programming languages, true is typically represented as 1 and false is represented as 0. Boolean variables are commonly used in programming to control the flow of a program, make decisions, and represent logical values.
Array
An array is a data structure that can store elements of the same data type. It is a fundamental concept in programming and is widely used to store data. In an array, each element can be accessed by an integer value indicating its position in the array. Arrays can be one-dimensional, two-dimensional, or multi-dimensional, depending on the number of indices required to access each element. They are widely used for tasks such as sorting, searching, and manipulating large sets of data.
Object
In programming, the object data type refers to a complex data type that represents a collection of data and functionalities. These serve as blueprints defining the characteristics and behaviour of the objects. An object contains data in fields or attributes, and methods that define its behaviour. The object data type is important because it allows programmers to design and implement complex systems by modelling entities as objects.
Tap to learn the PHP basics here- PHP variables and data types.
Types of variables in coding
In programming, variables can be classified into various types based on which data they store. Here are some common types of variables:
Local variables in programming.
Local variables are within a specific function or block. They are only accessible within that particular function or block. They are used to store temporary information as well as perform some calculations that are specific to that function or block.
Example:
function myFunction()
{
var x = 5; // Here, x is a local variable to the myFunction function
console.log(x);
}
Output: x=5
Global variables
Unlike the local variable, the global variable performs outside of any function or block and also can be accessible on the entire program and modified. It is advised to use limitedly.
Example:
Var globalVar=” I am a global variable”;
Function accessGlobalVar()
{
console.log(GlobalVar);
}
Output: I am a global variable
Constant variables in programming.
This type of variable is denoted by the keyword “const”. According to its name, it cannot be altered once the value is assigned. It is used for values that remain constant such as mathematical operations, and configuration values.
Example:
Const PI=3.14159;
console.log(PI);
Output: PI=3.14159
If we change the value of PI it will show an error because the value of PI is set constant as 3.14159
Dynamic variable
This type of variable is not commonly used in coding. It is not bound to a specific type and can assigned to different values. Dynamic variables allow flexibility but also have some complexities and potential runtime errors.
Tap to read about the leading technologies here- Top six fields.
Introduction to javascript programming data structure
JavaScript, a programming language, supports various data structures. These data structures play a crucial role in organising a program. Some of the commonly used data structures in JavaScript are:
Arrays
In JavaScript, the data structure arrays help to store multiple values in a single character. Those values can be numbers, alphabets and a mix of these values. They are kept between square brackets. This array data type is also known as zero-indexed since the values initiate from zero followed by 1 and so on.
Example:
let myArray=[1,2,3,4];
let fruits=[apple,strawberry,mango];
let mixedArray=[1,welcome,true];
An example of an array used to add elements
let myArray=[1,2,3];
myArray.push(4);
console.log(myArray);
Output
[1,2,3,4]
Objects
It is a collection of key-value pairs, where each key is a unique identifier or property name, and its corresponding value can be of any data type. Objects in JavaScript are widely used for representing real-world entities and for organizing data.
Example:
let person = new Object();
person.name = "Oscar";
person.age = 20;
person.email = "Oscar@example.com";
person.address = {
city: "Malmo",
country: "Sweden"
};
person.hobbies = ["reading", "hiking", "dancing"];
Maps
This programming data type stores the key values and remembers the order of keys. This is particularly useful for storing and managing data.
Linked Lists, Stacks, and Queues
These data structures are not built into JavaScript. They are implemented using arrays or objects to manage data in a specific manner.
Conclusion of variables and data types.
Variables in programming, store the data. Variables and data types in programming define which kind of data can be stored and operated on. Programming languages typically offer various programming variables and data types, such as numbers, strings, booleans, objects, arrays, and more, each serving individual purposes. Understanding and utilising different programming variables and data types is fundamental to effectively managing and processing data in programming.