In the realm of web development, creating interactive web pages is essential to engage users and provide dynamic experiences. One powerful tool for achieving interactivity is the HTML5 Canvas element combined with JavaScript. This duo empowers developers to craft visually stunning and interactive web pages directly within the web browser. In this article, we’ll delve into the fundamentals of leveraging JavaScript and HTML5 Canvas to build captivating web pages that respond to user actions.
How does HTML5 Canvas aids in building interactive web pages?
HTML5 Canvas is a powerful element that allows for dynamic interactive web pages, scriptable rendering of 2D shapes and bitmap images. It provides developers with a blank slate onto which they can draw graphics, charts, animations, and more using JavaScript. With Canvas, developers have fine-grained control over every pixel, enabling the creation of intricate visualizations and interactive elements.
To integrate a Canvas element into an HTML document, you simply include the <canvas> tag, specifying its width and height attributes. For instance:
<canvas id="myCanvas" width="800" height="600"></canvas>
Here, we’ve defined a Canvas element with an ID of “myCanvas” and dimensions of 800 pixels by 600 pixels.
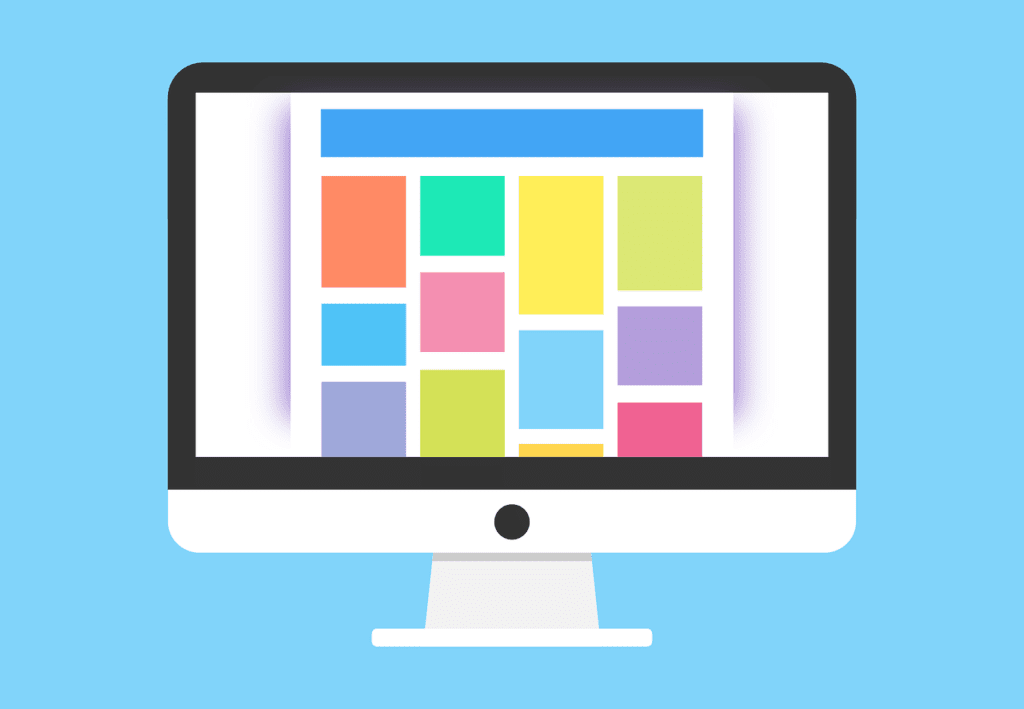
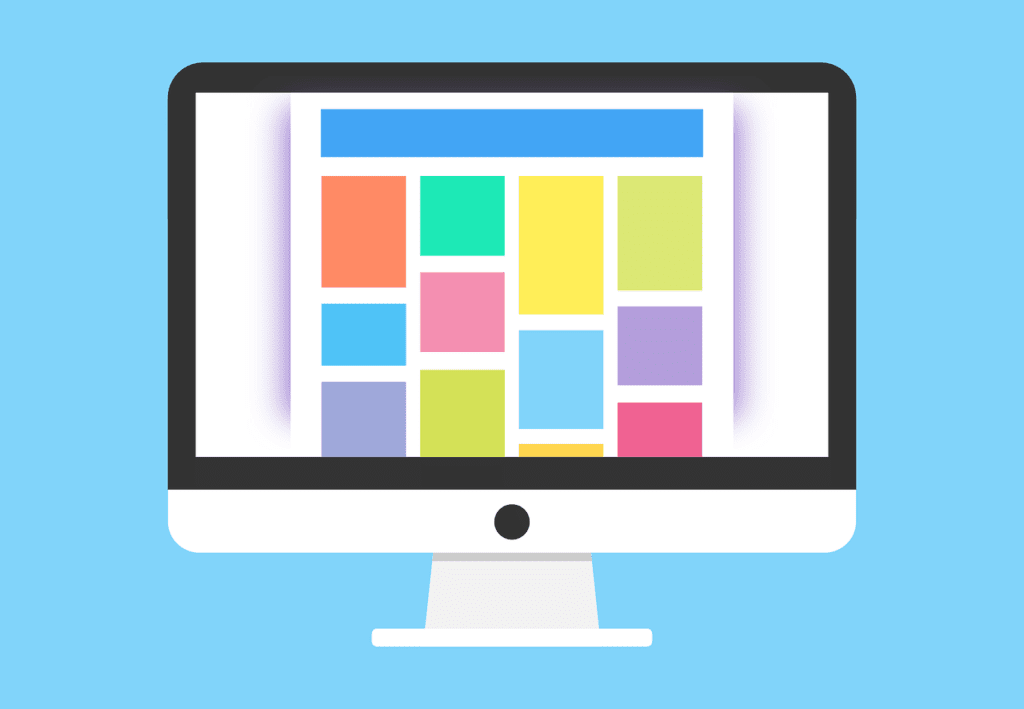
Getting Started with JavaScript:
JavaScript serves as the powerhouse behind Canvas-based interactivite web pages. With JavaScript, developers can manipulate Canvas elements, draw shapes, handle user input, and create animations that helps in making of interactive web pages.
To begin working with Canvas in JavaScript, you need to obtain a reference to the Canvas element in the HTML document. This is typically done using the getElementById method or other DOM traversal techniques. Once you have the reference, you can start drawing on the Canvas using various methods provided by the Canvas API.
Click to view our latest blog article on Javascript- JS best traits
Drawing on the Canvas:
The Canvas API offers a wide range of drawing methods to create shapes, paths, text, and images. Some commonly used methods for interactive web pages include fillRect(), strokeRect(), fillText(), strokeText(), arc(), lineTo(), and beginPath().
Let’s illustrate a simple example of drawing a rectangle on the Canvas using JavaScript:
const canvas = document.getElementById('myCanvas');
const context = canvas.getContext('2d');
// Draw a filled rectangle
context.fillStyle = 'blue';
context.fillRect(50, 50, 200, 100);
In this snippet, we first obtain a reference to the Canvas element with the ID ‘myCanvas’ and then get its 2D rendering context. We then set the fill style to blue and drew a filled rectangle at coordinates (50, 50) with a width of 200 pixels and a height of 100 pixels.
Adding Interactivity to web pages:
Interactivity breathes life into interactive web pages, making them more engaging and immersive. With JavaScript and Canvas, you can respond to user input such as mouse clicks, keyboard presses, or touch events to create interactive experiences.
Let’s enhance our previous example by making the rectangle draggable. We’ll enable users to click and drag the rectangle across the Canvas:
let isDragging = false;
let offsetX, offsetY;
canvas.addEventListener('mousedown', function(event) {
const rect = canvas.getBoundingClientRect();
offsetX = event.clientX - rect.left;
offsetY = event.clientY - rect.top;
isDragging = true;
});
canvas.addEventListener('mousemove', function(event) {
if (isDragging) {
const x = event.clientX - offsetX;
const y = event.clientY - offsetY;
context.clearRect(0, 0, canvas.width, canvas.height);
context.fillRect(x, y, 200, 100);
}
});
canvas.addEventListener('mouseup', function() {
isDragging = false;
});
In this modified code, we’ve added event listeners to track mouse events. When the user clicks inside the rectangle, we calculate the offset between the mouse pointer and the rectangle’s position. As the user moves the mouse, we update the rectangle’s position accordingly. Finally, when the user releases the mouse button, we stop dragging the rectangle.
Animating on the Canvas:
Animations enhance user engagement by adding motion and dynamism to interactive web pages. With JavaScript, you can create animations on Canvas by repeatedly redrawing the scene at specified intervals.
Let’s create a simple animation of a bouncing ball on the Canvas:
let x = canvas.width / 2;
let y = canvas.height / 2;
let dx = 2;
let dy = -2;
const radius = 20;
function drawBall() {
context.clearRect(0, 0, canvas.width, canvas.height);
context.beginPath();
context.arc(x, y, radius, 0, Math.PI * 2);
context.fillStyle = 'red';
context.fill();
context.closePath();
if (x + dx > canvas.width - radius || x + dx < radius) {
dx = -dx;
}
if (y + dy > canvas.height - radius || y + dy < radius) {
dy = -dy;
}
x += dx;
y += dy;
}
setInterval(drawBall, 10);
In this example, we define the ball’s initial position, velocity, and radius. We then repeatedly redraw the ball at specified intervals using the drawBall function. The ball bounces off the Canvas boundaries by reversing its velocity when it reaches the edge.
Click to learn about dashing design patterns- How to implement design pattern into your code.
Integrating User Input to build interactive web pages:
User input can greatly enhance interactivite web pages by allowing users to actively participate in the web experience. With JavaScript and Canvas, you can respond to various forms of user input, such as mouse clicks, keyboard presses, and touch events.
Let’s create a simple drawing application where users can draw on the Canvas using the mouse:
let isDrawing = false;
canvas.addEventListener('mousedown', function(event) {
const rect = canvas.getBoundingClientRect();
const x = event.clientX - rect.left;
const y = event.clientY - rect.top;
context.beginPath();
context.moveTo(x, y);
isDrawing = true;
});
canvas.addEventListener('mousemove', function(event) {
if (isDrawing) {
const rect = canvas.getBoundingClientRect();
const x = event.clientX - rect.left;
const y = event.clientY - rect.top;
context.lineTo(x, y);
context.stroke();
}
});
canvas.addEventListener('mouseup', function() {
isDrawing = false;
});
In this example, we track mouse events to determine when the user starts drawing, continues drawing, and stops drawing. As the user moves the mouse while holding down the mouse button, we draw a path on the Canvas using the lineTo method and stroke it to create a line.
Conclusion:
In conclusion, HTML5 Canvas combined with JavaScript offers a powerful platform for building interactive web pages that engage users and elevate the browsing experience. By mastering the fundamentals of Canvas drawing, incorporating interactivity, animating elements, and integrating with JavaScript libraries, developers can unleash their creativity and create compelling visualizations, games, simulations, and more.
With its versatility and flexibility, HTML5 Canvas remains a cornerstone technology for modern web development, empowering developers to bring their ideas to life on the web. Whether you’re a seasoned developer looking to enhance your skills or a newcomer eager to explore the world of web development, HTML5 Canvas and JavaScript provide an exciting playground for innovation and creativity.