Code readability is a fundamental aspect of software development that focuses on crafting source code in a way that is easily understandable by human beings. It is the art of crafting code in a clear and understandable manner. It involves adopting practices and conventions that enhance the clarity and comprehensibility of the code.
It also includes using consistent formatting, meaningful variable and function names and adding comments where necessary. Readable code is not only easier for others to comprehend but also facilitates collaboration among developers. By prioritizing code readability it contributes to maintainability, reduces the chances of errors and enhances the overall efficiency of the software development process.
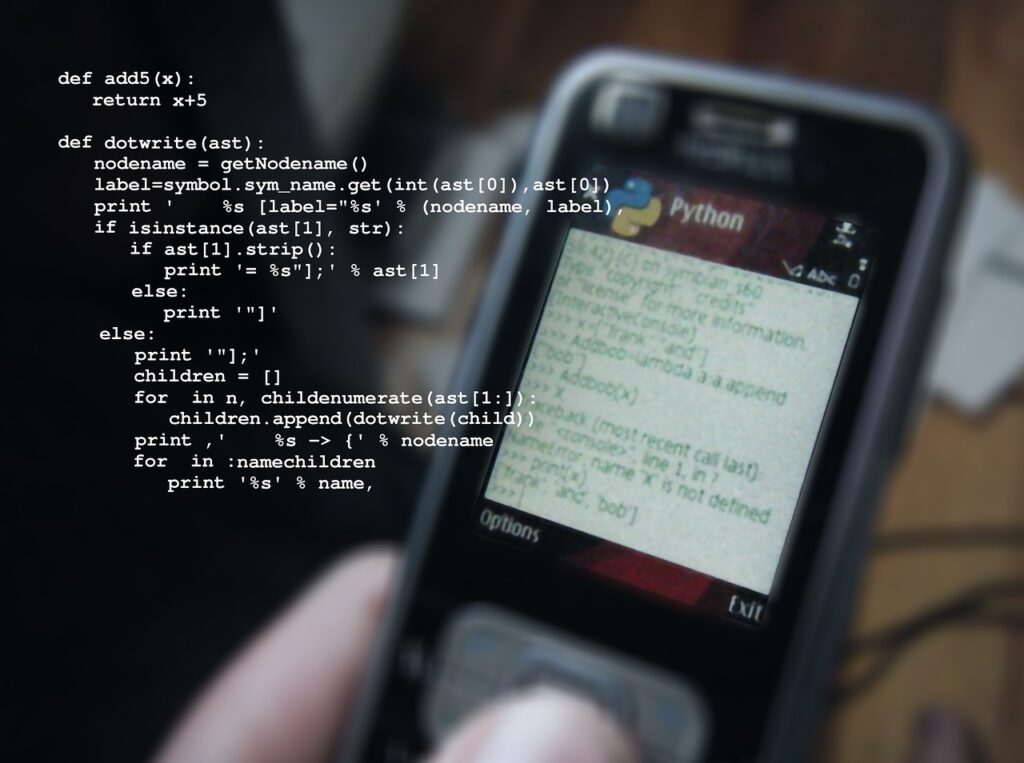
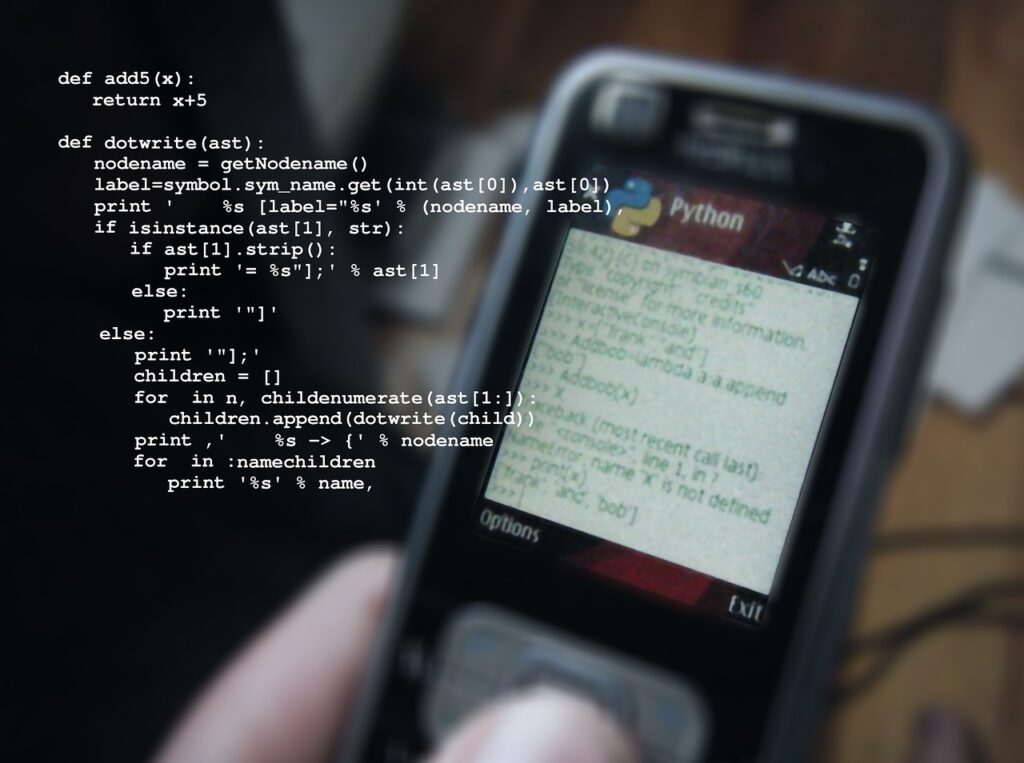
10 best practices for code readability
Code readability is crucial for maintaining, understanding and collaborating on software projects. Here are some best practices to enhance code readability:
1. Consistent Indentation;
Maintain uniform indentation throughout your work to enhance code readability and code documentation structure. This practice enhances readability by clearly indicating the hierarchy and nesting of different code sections such as loops, conditionals, and function definitions. Consistent indentation helps programmers understand the flow of the code at a glance, making it easier to follow and maintain.
2. Meaningful Variable Names
Use descriptive names for variables, functions and classes to convey their purpose without excessive comments. Instead of using generic or cryptic names, choose names that convey the purpose or content of the variable. This makes the code self-explanatory and helps other developers understand the role of each variable without delving into the code’s details. E.g. using “userInput” instead of “X” or “tempValue” can make your code more understandable and maintainable.
3. Consistent naming conventions:
Adhere to a consistent naming convention (E.g. camelCase or snake_case) to improve code consistency. Consistent naming in code involves using a uniform naming convention for variables, functions, and other elements throughout your codebase. This consistency makes it easier for developers to understand and navigate the code. It’s a good practice to establish naming conventions early in a project and adhere to them consistently across all parts of the code.
4. Modularization:
Break down complex tasks into smaller, modular functions or classes for easier understanding and maintenance. Each module focuses on a specific task or functionality, making the codebase more organized and easier to understand. The benefits of modularization include improved code readability, easier maintenance, and enhanced reusability of code segments. Modules can be independently developed, tested, and modified, promoting a more efficient and collaborative development process.
5. Comments for clarity:
Add comments sparingly, focusing on explaining complex sections or providing context rather than stating the obvious. Comments in code serve as annotations to explain complex parts, provide context, or clarify the purpose of specific lines or blocks of code. Using comments judiciously can enhance code readability. However, it’s crucial to strike a balance, avoiding unnecessary comments for obvious code and focusing on explaining non-intuitive aspects. Well-placed comments can help future developers understand the rationale behind certain decisions or the functionality of particular code segments.
Click to read our latest article on JS arrays- JS arrays speak better than words in programming.
6. Whitespace usage:
Use whitespace effectively to group related code and improve visual separation between different sections. Whitespace usage, including spaces and blank lines, is crucial for code readability. Properly incorporating Whitespace makes code visually clearer and easier to follow. Consistent indentation, appropriate line breaks, and spacing around operators contribute to a well-formatted and readable code style. Balancing whitespace effectively enhances the visual structure of the code, aiding in understanding code blocks, loops, and conditional statements.
7. Consistent formatting:
Follow a consistent code formatting style to create a uniform look across your codebase. This includes consistent indentation, spacing and naming conventions. Adopting and sticking to a particular coding style improves code readability and makes collaboration smoother among developers. Consistent formatting is often enforced through style guides or linters, ensuring that the entire codebase maintains a cohesive and organized appearance. This practice facilitates code reviews, debugging, and maintenance tasks.
8. Limit line length:
Keep lines of code within a reasonable length (commonly 80-120 characters) to prevent horizontal scrolling and enhance readability. Shorter lines also make it easier to compare code side by side or view multiple files simultaneously. This practice encourages more concise and focused code, contributing to improved maintainability and collaboration among developers. Many coding style guides recommend adhering to specific line length limits to promote consistency across the codebase.
9. Logical flow:
Organize code in a logical sequence, making it easy for others to follow the flow of execution. Logical flow in code refers to the sequential order and organization of statements to achieve a clear and understandable progression of operations. A well-designed logical flow ensures that the code follows a natural structure, making it easier to comprehend. This involves organizing functions, loops, and conditional statements in a way that aligns with the intended logic of the program. A logical flow promotes code readability, aids in debugging, and facilitates collaboration by allowing developers to follow the sequence of actions in a straightforward manner.
10. Error handling:
Implement clear and concise error handling to provide helpful information in case of issues and make debugging easier. Effective error handling contributes significantly to code readability by providing clarity on how the program deals with unexpected situations. When error-handling mechanisms are appropriately integrated into the code, it becomes evident how the program responds to potential issues. This transparency aids developers in understanding the resilience of the code and allows them to follow the logic even when errors occur.
By incorporating these practices, you can create code that is not only functional but also easily understandable and maintainable.
Improve code readability
To improve code readability, consider the following practices.
- Meaningful names- Use descriptive and meaningful names for variables, functions, and classes.
- Consistent formatting- Follow a consistent coding style, including indentation, spacing, and naming conventions.
- Proper Indentation- Indent code blocks consistently to represent the logical structure of the program.
- Limit line length- Keep lines of code within a reasonable length to prevent horizontal scrolling.
- Whitespace usage- Use whitespace effectively to enhance visual clarity and separate code blocks.
- Comments for explanation- Add comments to explain complex parts of the code, provide context, or clarify intentions.
- Logical Flow- Organize code in a logical and sequential manner to improve the flow of understanding.
- Error handling- Implement clear and effective error-handling mechanisms with informative error messages.
- Modularization- Break down large and complex code into smaller, focused modules or functions.
- Consistent naming- Apply consistent naming conventions for variables, functions and other elements.
- Documentation- Provide comprehensive documentation for functions, classes, and modules.
- Remove redundancy- Eliminate redundant code or unnecessary comments that don’t add value.
- Use whitespace to group related code- Group related code together with blank lines to visually separate different sections.
- Consider code reviews- Engage in code reviews to gather feedback on readability and coding practices.
- Stay mindful of the complexity- Be mindful of code complexity; consider breaking down complex logic into simpler functions.
Applying these practices consistently can significantly enhance the readability of your code, making it more understandable and maintainable for both you and other developers.
Click to view the trendy article on how to avoid common coding mistakes.
How to do document a code.
Documenting code involves adding comments, explanations, and other forms of text to the source code to provide additional context, clarification, or instructions. This documentation serves as a guide for developers, making it easier to understand and maintain the codebase.
Documenting code is essential for several reasons:
Understanding: It helps developers understand the purpose and functionality of the code.
Maintenance: Well-documented code is easier to maintain and update, especially by developers who did not originally write the code.
Collaboration: It facilitates collaboration within a team, enabling team members to work more effectively together.
Onboarding: New team members can quickly get up to speed with the project by referring to the documentation.
Debugging: Comments can provide insights into potential issues or offer suggestions for debugging.
Reuse: Proper documentation makes it easier to reuse code in other projects.
Documenting code is crucial for maintaining readability and facilitating collaboration.
Best practices for documenting code.
Use meaningful comments
•Write comments that explain the purpose and intent behind the code.
Follow a documentation standard
•Consistently apply a documentation style across your codebase for a cohesive look and feel.
Write clear function and method signatures
•Includes descriptive and clear explanations of parameters, return values, and the purpose of functions and methods.
Use docstrings
•Utilize docstrings for the functions, classes, and modules to provide inline documentation.
•Choose a standard format for your docstrings, such as reStructuredText or Markdown.
Update documentation regularly
•Keep documentation synchronized with code changes. Outdated documentation can lead to confusion.
Explain code design choices
•Document the reasoning behind significant design decisions to provide context for future developers.
Provide examples
•Include usage examples to illustrate how to interact with and use functions or classes.
Document dependencies
•Clearly specify external dependencies, versions, and any installation or setup instructions.
Include a README file
•Create a comprehensive README file that serves as a guide for users and developers, covering project overview, setup instructions, and usage details.
Use version control messages wisely
•Write informative commit messages that explain the purpose and impact of code changes.
Consider inline comments sparingly
•Use inline comments only when necessary. Prioritize writing self-explanatory code over excessive comments.
Include license information
•Clearly state the license under which the code is released to communicate legal usage terms.
Collaborate on documentation
•Encourage team collaboration on documentation to ensure a collective understanding and maintenance.
Separate concerns
•Keep code and documentation separate. •Avoid cluttering the code with excessive comments or documentation.
Accessibility
•Ensure that your documentation is accessible and understandable by developers with varying levels of expertise.
Conclusion
By adhering to these best practices, you create a good code readability and code documentation that not only aids in understanding the code’s functionality but also fosters a collaborative and maintainable development environment.