In the dynamic and ever-evolving field of software development, the practice of code optimization holds a place of paramount importance. It’s a critical factor that greatly influences the performance, efficiency, and overall reliability of software applications. This article aims to delve deep into the complex and nuanced world of code optimization, shedding light on the myriad of ways in which developers can fine-tune their code for optimal performance.
The process of code optimization is multifaceted and involves a careful balance of various techniques and strategies. At its heart, it’s about enhancing the speed at which a program operates and reducing the resources it consumes, such as memory and processing power. This is not just about tweaking and adjusting code; it’s a strategic approach to rethinking and restructuring how a program works to make it as efficient as possible.
Code optimization is an essential aspect of software development, aiming to enhance the performance of a software system. This process involves a strategic modification of the code to ensure it operates more efficiently, whether that means executing tasks faster or consuming fewer resources like memory and processing power. At its core, code optimization is about achieving maximum efficiency in software execution without unnecessarily straining the system’s resources.
Basics of code optimization
The fundamental principle of code optimization is to identify and address the ‘bottlenecks’ or the most significant inefficiencies in the code. Bottlenecks can manifest in various forms, such as unoptimized loops, inefficient algorithms, or redundant processes unnecessarily consuming system resources. By pinpointing these areas, developers can focus their efforts on parts of the code that will yield the most significant performance improvements when optimized.
However, it’s crucial that in the pursuit of optimization, developers do not compromise on the code’s readability and maintainability. Readability refers to how easily other developers can understand the code, a vital factor for collaborative projects and future maintenance. Maintainability, on the other hand, involves the ease with which the code can be updated, fixed, or expanded. Optimizations that overly complicate the code, making it hard to understand or maintain, can be counterproductive in the long run.
They may introduce new bugs, make it difficult to implement future changes, or cause issues when the code interacts with other software components. Effective code optimization strikes a balance between improving performance and preserving, or even enhancing, the code’s readability and maintainability. This approach ensures that the software runs efficiently and remains adaptable, scalable, and accessible for ongoing development efforts. Here are some tips and tricks:
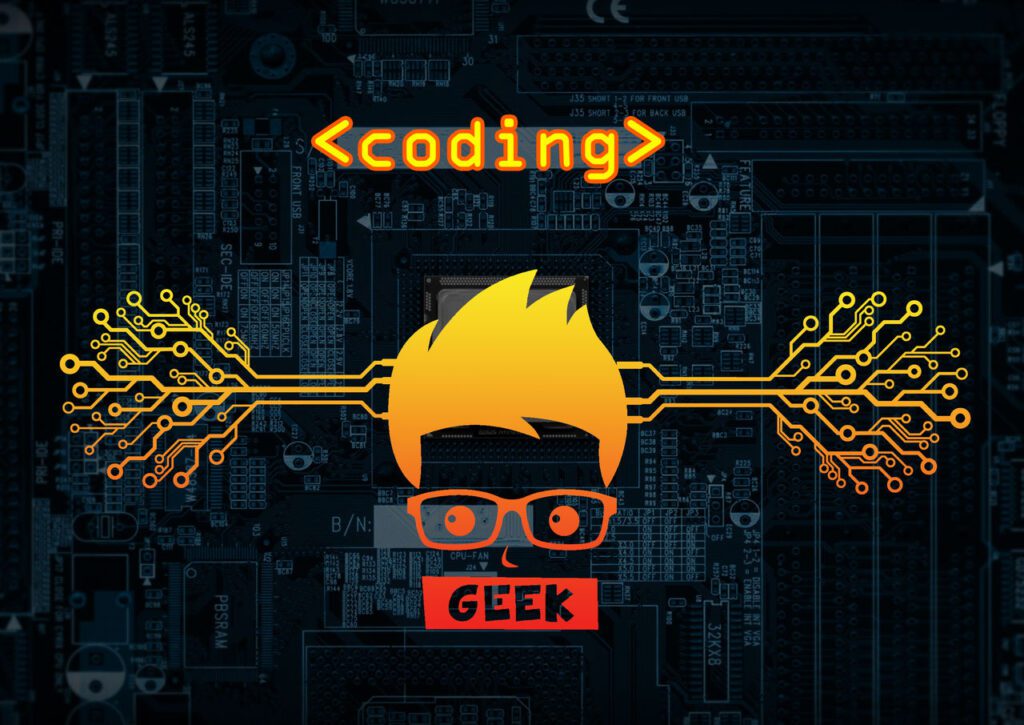
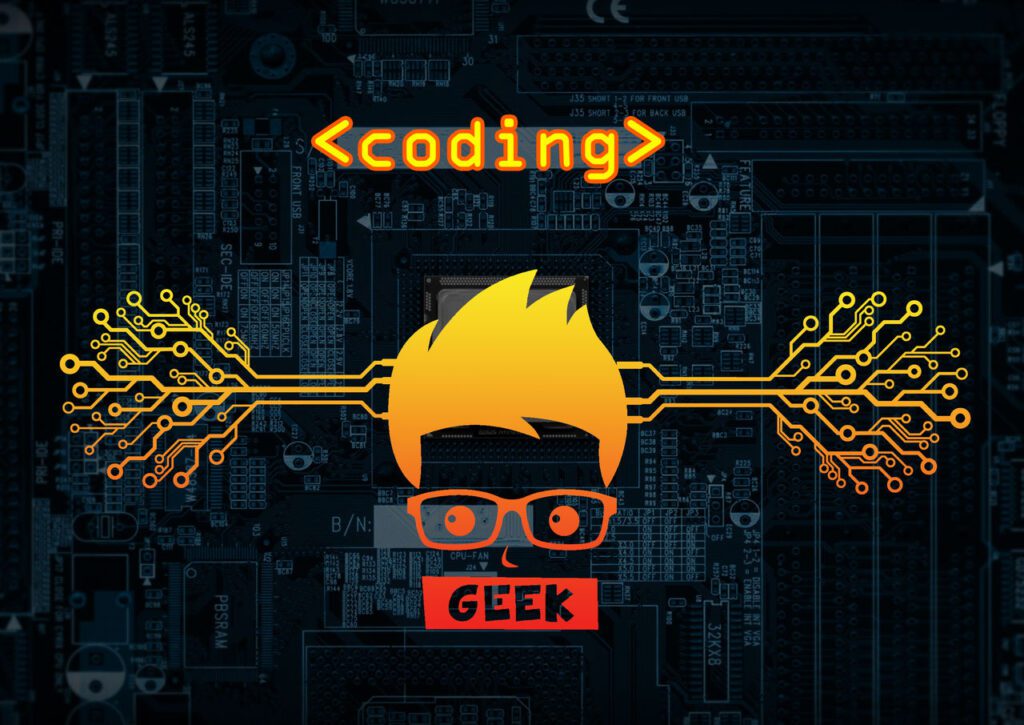
Performance Tuning Tips for code optimization
1. Profiling Before Optimising:
Before diving into code optimization, it’s imperative to identify where the actual performance issues lie. This is where profiling comes into play. Profiling tools are designed to analyze your application’s performance, providing detailed insights into various aspects like memory usage, CPU time, and execution frequency of different code segments. By using these tools, developers can pinpoint the slowest parts of their code, known as ‘bottlenecks’. This targeted approach ensures that optimization efforts are focused and effective, rather than based on guesswork. Profiling allows developers to make data-driven decisions about where to concentrate their optimization efforts for maximum impact.
2. Optimise Algorithms:
The choice of algorithms and data structures plays a crucial role in the performance of an application. In many cases, performance issues can be significantly mitigated or even solved by choosing more efficient algorithms. For instance, switching from a brute-force approach to a more sophisticated algorithm can reduce processing time from hours to minutes. Similarly, choosing the right data structure can dramatically improve the efficiency of data access and manipulation. Developers should continually evaluate and reconsider their algorithmic choices, especially in performance-critical sections of the application, to ensure they are using the most efficient approach possible.
3. Avoid Premature Optimization:
A common pitfall in software development is premature code optimization – the practice of trying to optimize code too early in the development process. This often leads to complex, hard-to-read, and maintainable code, which might not even address the real performance issues. The key is to first focus on building functional and reliable code, ensuring that the application works correctly. Once the functionality is in place and performance bottlenecks have been identified through profiling, targeted optimization can be more effectively applied. This approach not only leads to better-optimized code but also maintains the readability and maintainability of the codebase.
Software Efficiency Techniques
Click to read our latest article on Responsive web design-
1. Code Refactoring:
Code refactoring is the process of restructuring existing computer code without changing its external behavior. Its primary goal is to improve the nonfunctional attributes of the software. Regular refactoring helps in cleaning up the codebase, making it more efficient and easier to understand, maintain, and extend. This process involves reorganizing code, optimizing algorithms, simplifying decision structures, and removing redundant or obsolete code. By doing so, refactoring can often lead to improved code performance as more efficient patterns replace less efficient ones. Moreover, well-refactored code is easier to debug and test, which indirectly leads to more efficient software development processes.
2. Memory Management:
Efficient memory management is crucial in software efficiency of code optimization. It involves properly allocating, using, and deallocating memory in applications. Efficient memory management helps in minimizing memory leaks and other issues that can slow down or even crash a software application. This includes deallocating unused memory, avoiding memory leaks, and using memory-efficient data structures. For high-performance applications, especially those running in resource-constrained environments like mobile devices, efficient memory management can significantly enhance overall application performance and responsiveness.
3. Parallel Processing:
Parallel processing refers to dividing a program into multiple components that can run simultaneously, typically by using multi-threading or deploying the software across multiple processors. This approach is particularly beneficial for performance-critical applications or those dealing with large data sets or complex computational tasks. By utilizing multi-threading and parallel processing, tasks that were previously executed sequentially can be processed concurrently, drastically reducing the overall execution time. This is especially relevant in an era where multi-core processors are ubiquitous. However, parallel processing requires careful design to avoid issues like race conditions and to ensure thread safety. When implemented correctly, it can lead to significant improvements in software execution speed.
Code Speed Improvement
1. Loop Optimization:
Loops are fundamental constructs in programming but can become sources of significant inefficiency, especially when they handle intensive tasks or manage large datasets. Optimizing loop conditions and minimizing the work performed inside loops can dramatically increase execution speed. This can involve several tactics:
– Minimizing the complexity within loops: Ensuring that the code inside the loop is as simple and streamlined as possible. This might mean moving calculations or conditional statements outside the loop if they don’t need to be repeatedly executed.
– Reducing loop overhead: For instance, using efficient loop constructs and minimizing the use of function calls within loops.
– Eliminating unnecessary loop iterations: This can be achieved by refining loop conditions or employing break statements to exit the loop as soon as its purpose is fulfilled.
2. Use of Efficient Libraries:
Selecting and utilizing well-optimized libraries can significantly enhance code performance. Libraries, especially those that are widely used and well-maintained, often undergo extensive optimization by the community or maintainers. They can provide efficient implementations of algorithms and data structures that would be time-consuming and complex to develop independently. By leveraging these libraries, developers can not only save development time but also boost code performance due to the sophisticated optimizations these libraries implement.
3. Minimizing I/O Operations:
Input/output operations are typically slower than CPU operations, especially when they involve disk access or network communication. Minimizing these operations can therefore lead to faster code execution:
– Optimizing the number of I/O calls: Batch processing or combining I/O operations can reduce the overhead associated with these operations.
– Caching: Storing frequently accessed data in a faster access memory location can reduce the need for repeated I/O operations.
– Asynchronous I/O: In cases where I/O operations are unavoidable, using asynchronous I/O can allow the program to continue executing other tasks while waiting for the I/O operation to complete, thereby improving the overall speed and responsiveness of the application.
Learn know-how about the efficient time management techniques for programmers.
Programming Optimization Strategies
1. Understanding Compiler Optimizations:
Compilers play a crucial role in code optimization. They come with a variety of built-in optimizations that can significantly enhance performance by transforming and streamlining code during the compilation process. Understanding these optimizations is key for developers, as it allows them to write code in a way that maximizes the efficiency of these compiler-level enhancements.
– Learn about specific compiler optimizations: Different compilers offer various optimization features, such as loop unrolling, inline expansion, and dead code elimination. Familiarize yourself with the optimizations available in your compiler and how they affect code execution and performance.
– Write compiler-friendly code: Certain coding practices can either aid or hinder compiler optimizations. For instance, avoiding overly complex expressions and maintaining a modular code structure can help the compiler more effectively optimize your code.
– Use compiler flags and settings: Most compilers allow you to customize optimization settings. Experiment with these settings to see how they affect your application’s performance, but be mindful of the trade-offs, as aggressive optimization can sometimes lead to less readable code or obscure bugs.
2. Benchmarking:
Benchmarking is the process of measuring the performance of your code against specific metrics. It is a critical part of the optimization process, as it provides quantifiable data on how well your optimizations are working.
– Establish performance metrics: Determine what performance metrics are most relevant to your application, such as execution time, memory usage, or CPU utilization.
– Use benchmarking tools: Utilize tools and libraries designed for benchmarking to measure your code’s performance consistently and accurately.
– Regularly benchmark: Continuously benchmark your code throughout the development process, especially after making changes or optimizations, to ensure that these alterations are having the desired effect on performance.
3. Learning and Applying Best Practices:
Staying informed about the latest programming best practices is vital for continual improvement and optimization of your codebase.
– Stay updated with the latest developments: The field of software development is constantly evolving. Keep abreast of the latest trends, techniques, and tools in coding and optimization.
– Apply best practices in coding: Implement best practices such as writing clean, readable, and maintainable code. This not only makes your code more efficient but also more accessible to other developers.
– Learn from the community: Engage with the developer community through forums, social media, and professional networks. Sharing knowledge and experiences can provide valuable insights into effective optimization strategies.
Conclusion
Code optimization is an ongoing process in the journey of software development. By implementing these performance tuning tips, software efficiency techniques, and programming optimization strategies, developers can significantly enhance the speed and efficiency of their applications, leading to more robust and reliable software solutions.